By Carmel Schvartzman
In this tutorial we'll learn how to get data via Ajax using jQuery, an Action method and a PartialView in Asp.Net MVC.The data for this application will be stored in an XML file, and on clicking a button, an Ajax request will be send from the jQuery function $.get () , to the web server. This latter will handle the HTTP-GET request inside an Action method, which in turn will respond sending the contents of a PartialView as HTML string, which will be displayed in the View as follows :
The XML file is the following:
<?xml version="1.0" encoding="utf-8" ?><notes> <note> <to>Fry</to> <from>Leela</from> <heading>Reminder</heading> <body>Don't forget me this weekend!</body> </note> <note> <to>Leela</to> <from>Fry</from> <heading>Finally!!!</heading> <body>Leela, finally you accept to have a date with me!</body> </note> <note> <to>Fry</to> <from>Leela</from> <heading>Rejection</heading> <body>You know what? In second thoughts, i'll go out with someone else...</body> </note></notes>
First we'll need a Controller to handle the Ajax requests, so build it with the code:
public class NotesController : Controller { public ActionResult Index() { return View(); }Next add a View to display just a button and a placeholder for the Ajax request results :
@{ ViewBag.Title = "Index";}
<h3>Using the jQuery $.get() requesting data from an MVC Action method</h3><div class="container"> <div id="results"></div> <button id="btn-action">Get data from Action method via Ajax</button>
</div><script src="~/Scripts/Notes.js"></script>
Just check - and fix it if it's necessary - that you feed this View with all the proper scripts and stylesheets coming through the _Layout page :
Then add the following CSS3 , to get the style of the web page :
.container { width: 80%; background: #eee; margin: 20px auto; padding: 30px; border: 1px solid #ccc; border-radius: 10px; box-shadow: 10px 10px 1px #c0c0c0; transition: all 3s ease-out 0.5s; text-align: center;}.note { width: 33%; background: #ddd; margin: 20px auto; padding: 30px; border: 1px solid #eaeaea; border-radius: 10px; box-shadow: 10px 10px 1px #c0c0c0; transition: all 3s ease-out 0.5s; text-align: center;}.note:hover { -webkit-transform: rotate(-1deg) scale(1.1,1.1);}button { border-radius:10px;}
Now for the script, when the button is clicked, we send an Ajax request to the web server :
$(function () { $("button[id$=action]") .button() .click(function (event) { event.preventDefault(); $.get("/Notes/GetAllNotes", null, function (res) {
$("#results").html(res);
}); });});
First we code event.preventDefault() for the button to unbind any behavior like "submit" or another onclick handler that could be defined.
Then we send an HTTP-GET request to the server. Because the web server will send an text/html response, which proceeds from a PartialView template, we just insert that HTML inside a <div> using the jQuery .html() method, which in turn calls the javascript .innerHTML property of the DOM element.
On the Controller, we add the method to handle the HTTP-GET request:
public ActionResult GetAllNotes() { XDocument xml = XDocument.Load(Server.MapPath("~/App_Data/Notes.xml")); return PartialView("_NotesList", xml); }The PartialView returned will get the XDocument as Model, and loop over the "note" elements , displaying each one inside a <div> :
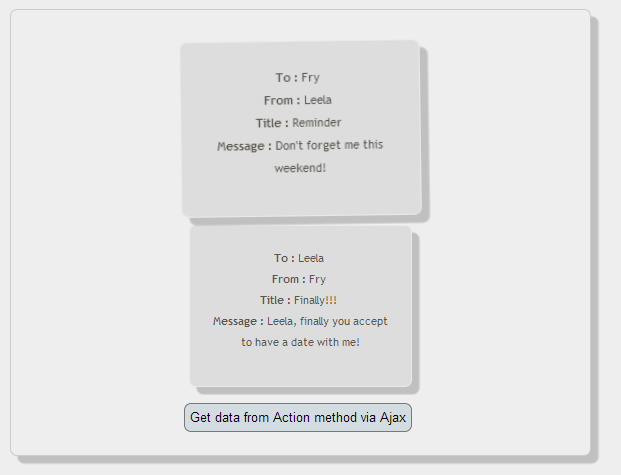
@model System.Xml.Linq.XDocumentWe just make use of the .Descendants( "" ) and the First().Value properties, to get the data to be displayed. Each Note node will be displayed as shown here :
@foreach (var note in Model.Descendants("note")){ <div class="note">
<p><b>To : </b><span>@note.Descendants("to").First().Value </span> <br /> <b>From : </b><span>@note.Descendants("from").First().Value </span> <br /> <b>Title : </b><span>@note.Descendants("heading").First().Value</span><br /> <b>Message : </b><span>@note.Descendants("body").First().Value </span></p>
</div>}
The web page will show initially just the button:
And when clicked, it will display the data:
That's all!! In this tutorial we've learned how to get data via Ajax using jQuery, an Action method and a PartialView in Asp.Net MVC.
Happy programming.....
כתב: כרמל שוורצמן
No comments:
Post a Comment